Bare Bones (Client-side Application)
Flavor: React
As a reminder, here's a link to how this series will unfold and a guide to setting up your dev environment (coming soon).
To get started, we go into our terminal program and run a command that will haunt your dreams after a while:
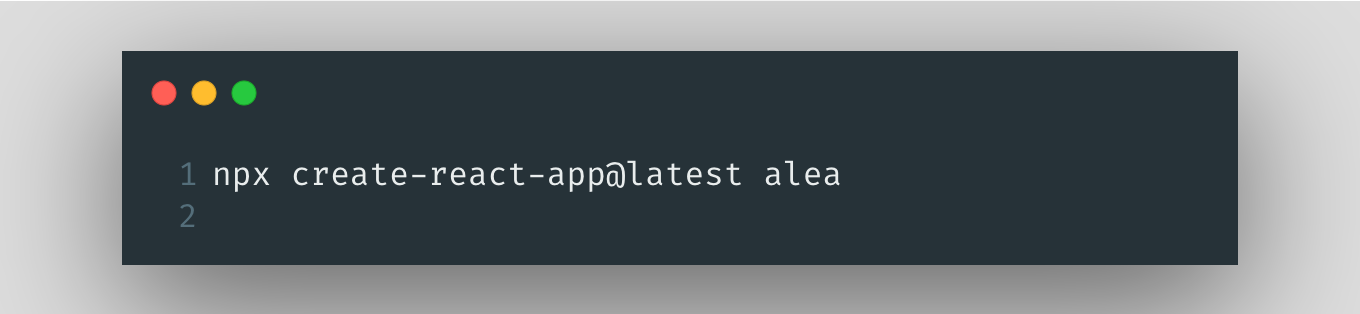
This command will go through all of the installation of packages necessary to get a project up and running, and create the project folder under the name that you gave it. At the end of the install, it gives you some options for commands to run - go ahead and npm start
in the console. It'll clear, and you should see that your React app is running happily on localhost:3000
. If we go there using our web browser: DISCO! We're in business.
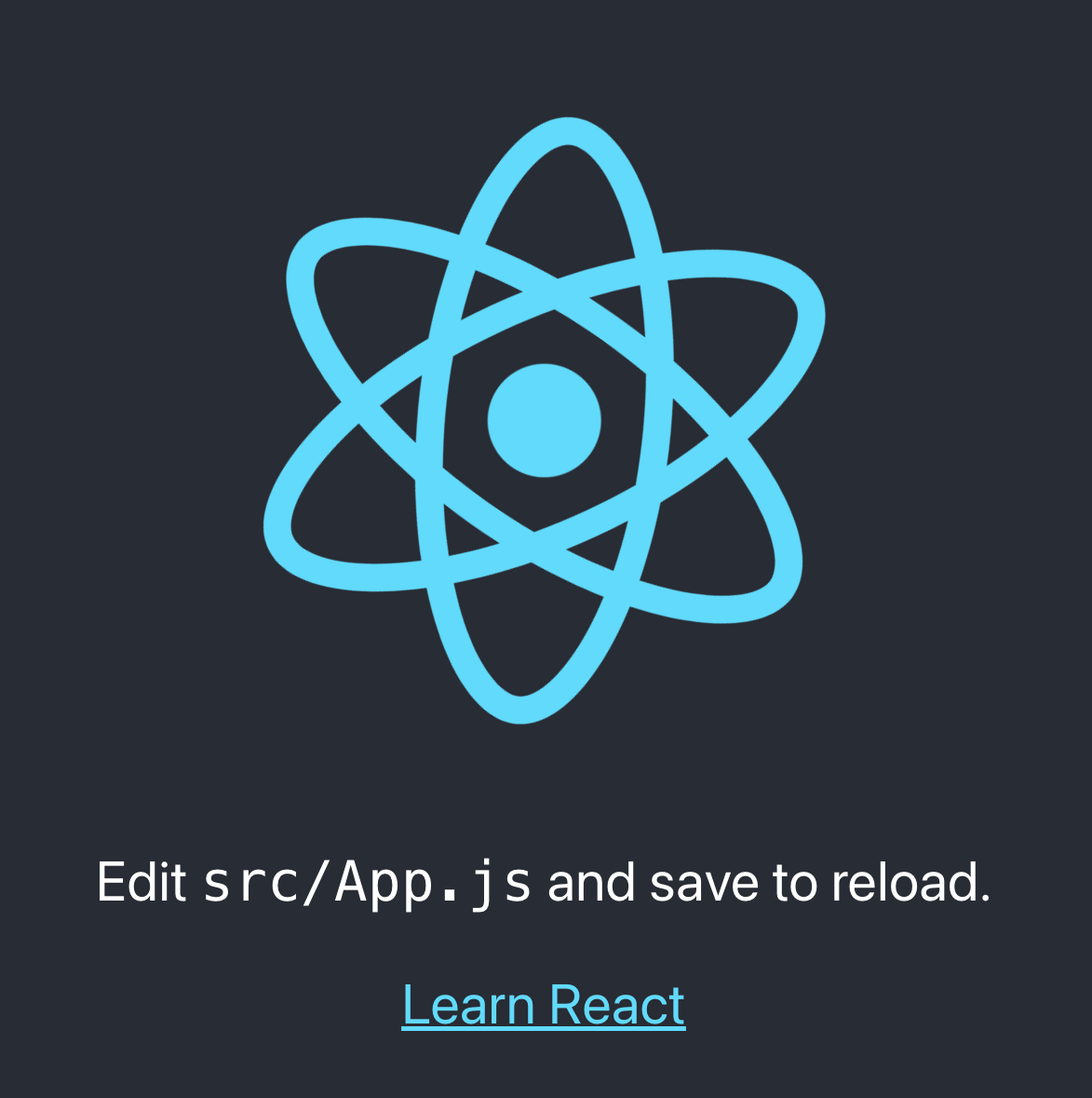
It's not much, but hey, it's yours! You made it happen. If this is your first time doing any coding: Congratulations, you've already done the thing! You can stop RIGHT HERE and I guarantee you that you can look someone in the eye at a party and tell them you've started doing web development.
Beyond the Boilerplate
Now go to your IDE of choice and open the folder you created. You'll see a file structure that looks something like this:
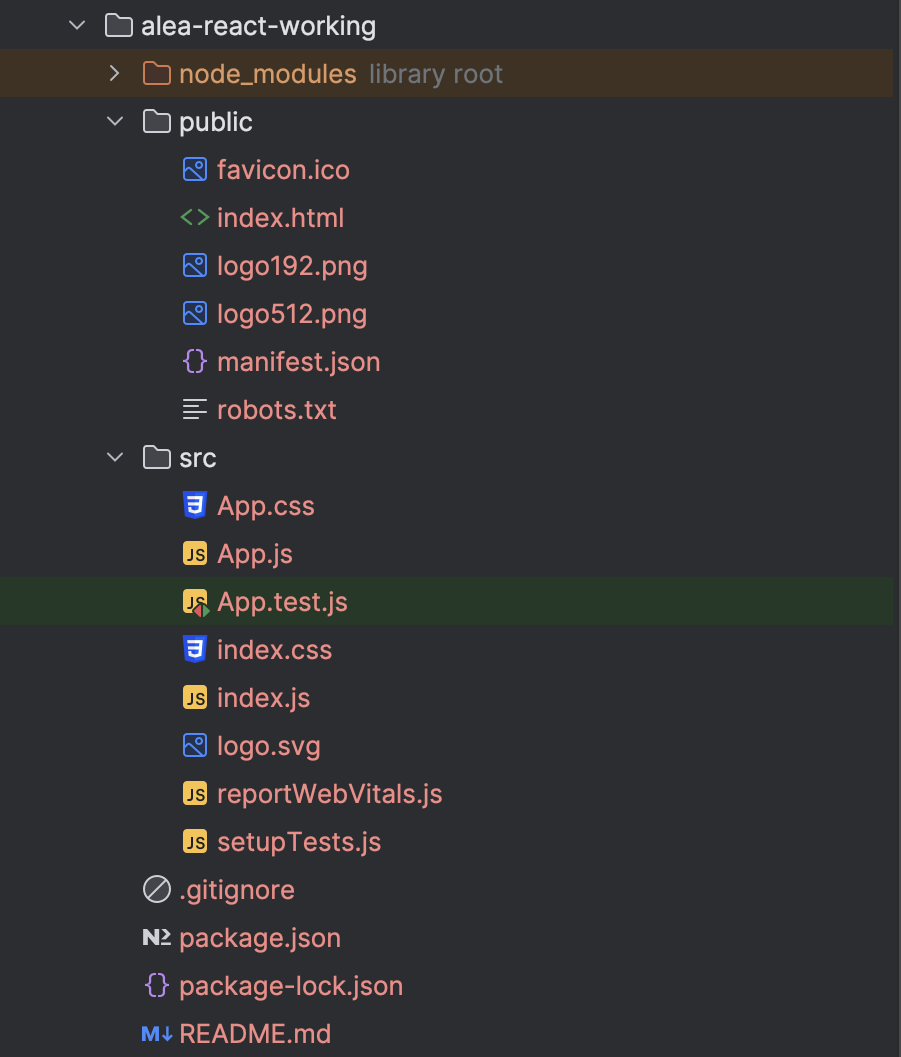
Even for something as simple as the page we just looked at, there's a lot of stuff that happens under the hood, and at some point or another, you might touch all of these files or, at the very least, understand what they are and why they're important. For now, we'll start by opening src/App.js
, which has the code that makes up the page we saw on port 3000.
As a reminder, all code in this series is given in screenshot form. No copy-pasting!
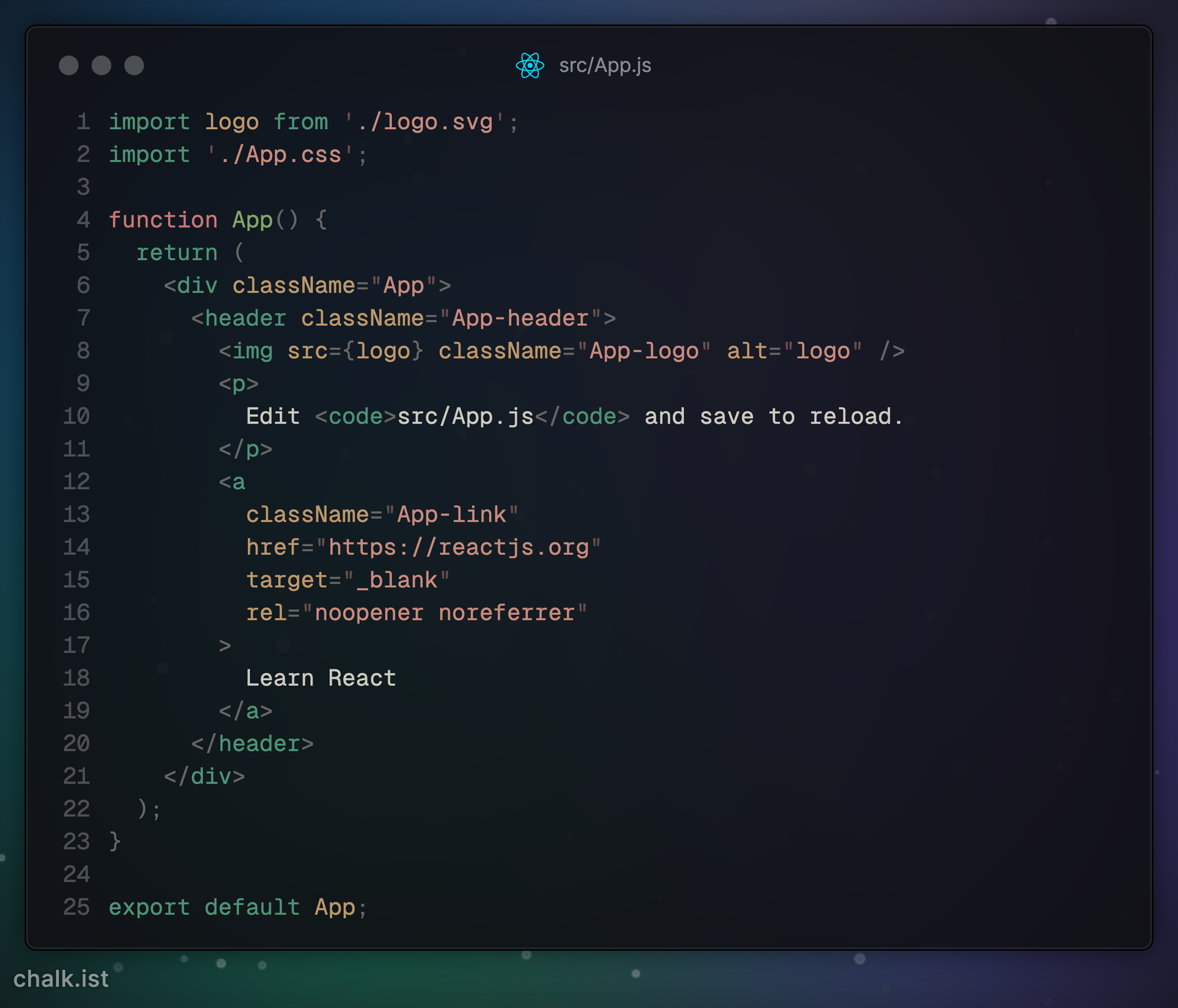
If this looks intimidating, take a few square breaths, then let's look at the three essential parts:
- There are the
import
statements that load in the spinning logo image and the other styling for the page. - A function
App
whose only job is to return the JSX (JavaScript eXtension), which is just a blend of Javascript and HTML that is compiled by the React library to make the final HTML we see in the browser. - The
export
statement for theApp
function. By exportingApp
, we make it available to the rest of our code. In this case, if you opensrc/index.js
, you'll see the JSX tag corresponding to the exportedApp
here.
Even in this straightforward file, you can see the whole loop that makes up React: we import things we need, declare a function to make a piece of HTML that we want, and then export it to be used somewhere else. Everything we do after this adds detail and decoration to this process.
Speaking of which, let's start by scooping out the template JSX in function App ( )
for our dice roller. We need:
- A title so people know the name of our app. (BRANDING!)
- An input for the number of dice to roll.
- An input for the type of dice to roll.
- A button to make everything GO.
- An output area with the results from the input.
For now, we won't worry about anything WORKING, although some of it will 'work' automatically. Without doing anything fancy, we get this:
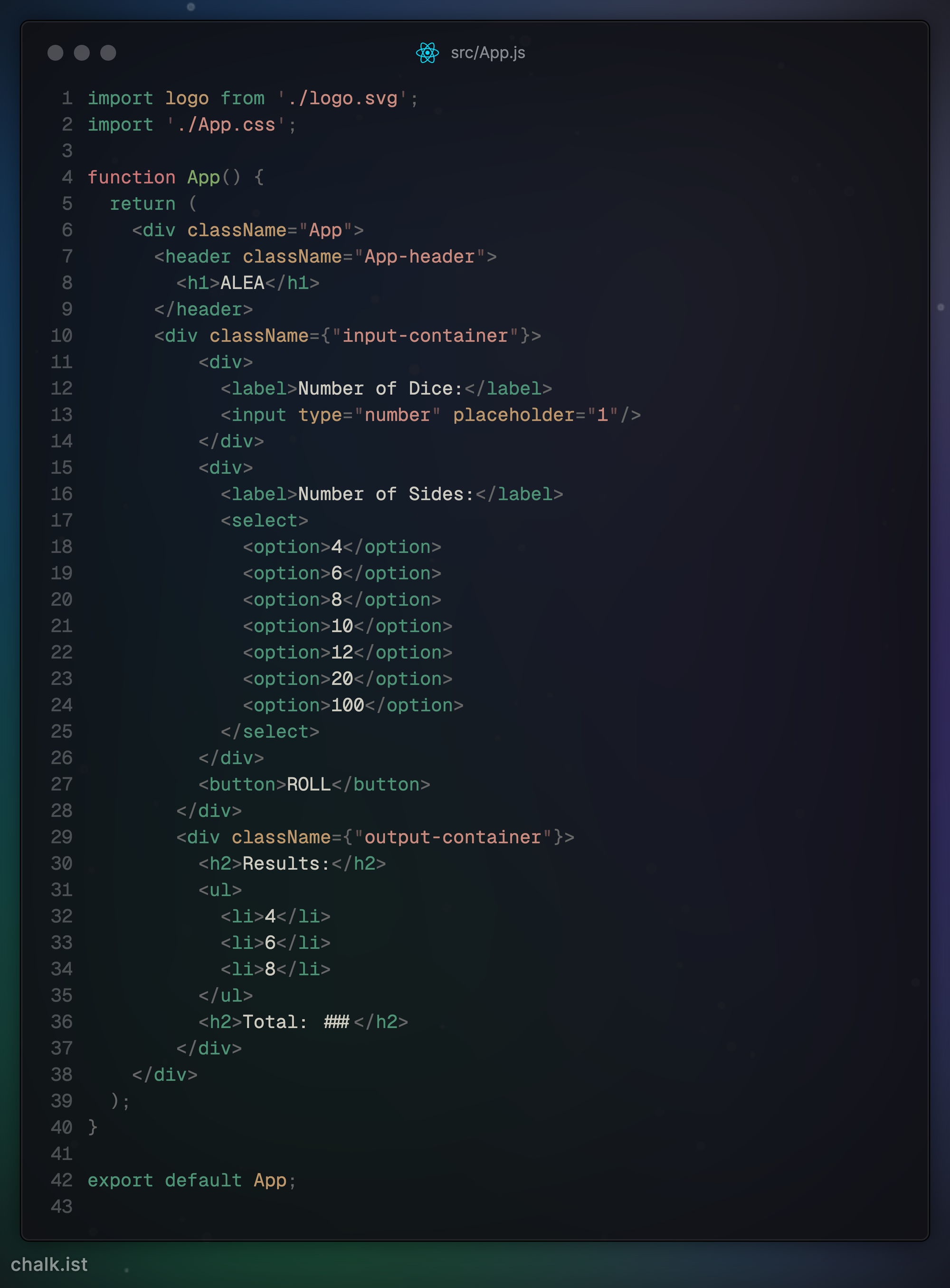
Once you're done, you can go back to the browser and behold the majesty of what you've made.
Breaking Shit
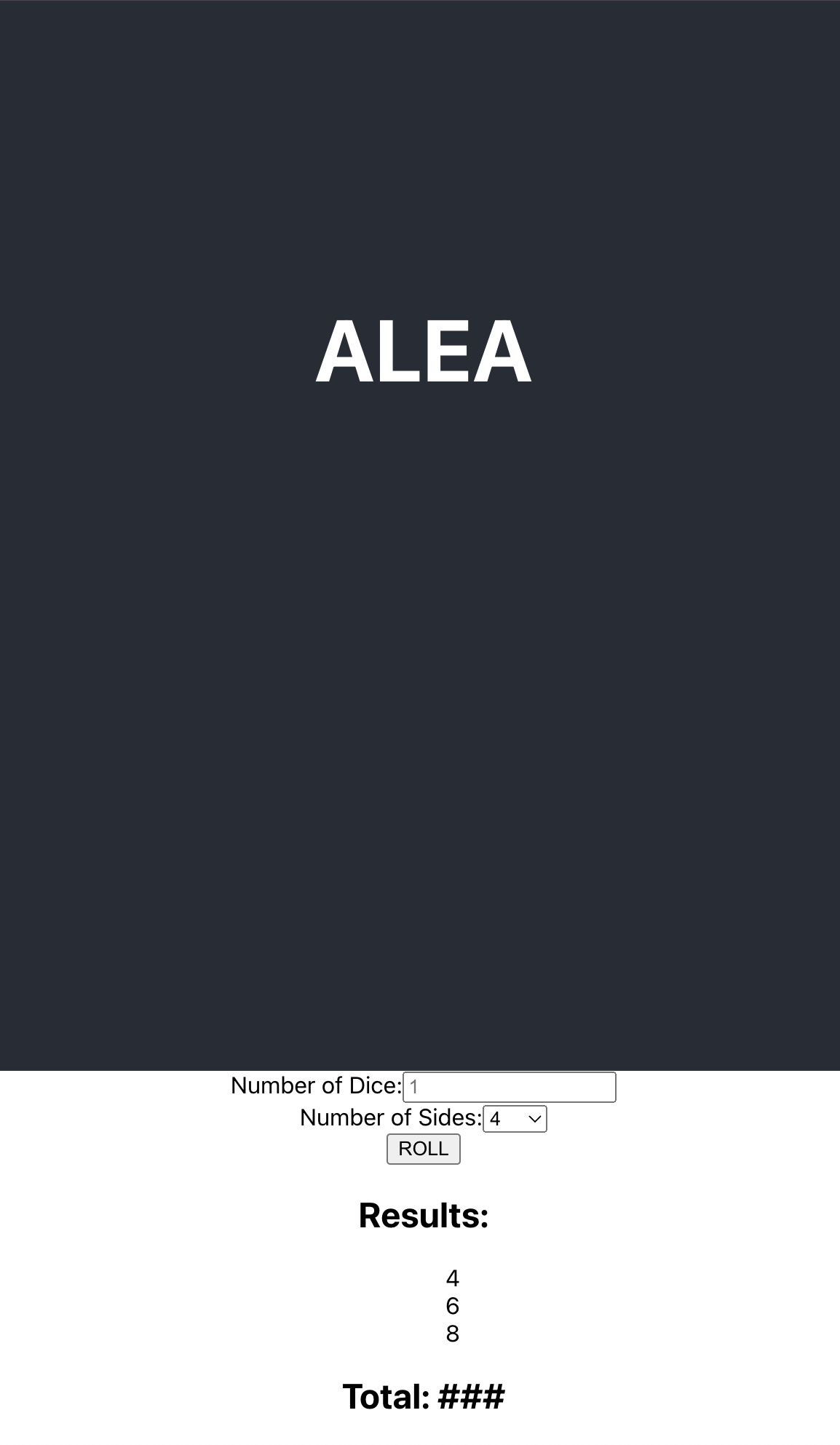
Congratulations. You've officially Broken the Shit out of something you're working on, which is a time-honored tradition in development. The whole process is to start with something simple, break the shit out of it to get it closer to what you want it to be, fix all the broken bits, and wind up with something new that you'll get to break all over again.
You'll also notice in your console that React isn't happy with us either:
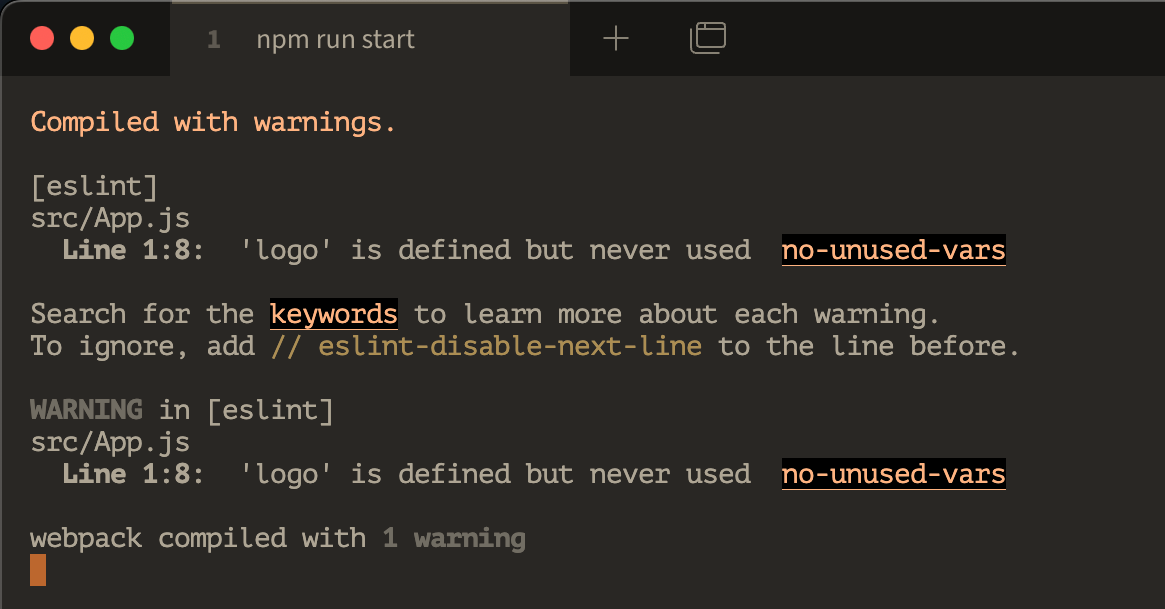
This is an easy fix - remove the import logo
statement from the beginning of the file and save it again. Voila! Typically, this is how it works: I fix things in the console first, since those will eventually stop everything from building, leaving you looking at Javascript errors. Then, I fix all the visual problems and get things back to looking pretty. (Note: In this case, we're just going to concentrate on getting things LEGIBLE, with minimal fuss.) To do that, we go into the App.css
and replace the contents with the CSS below.
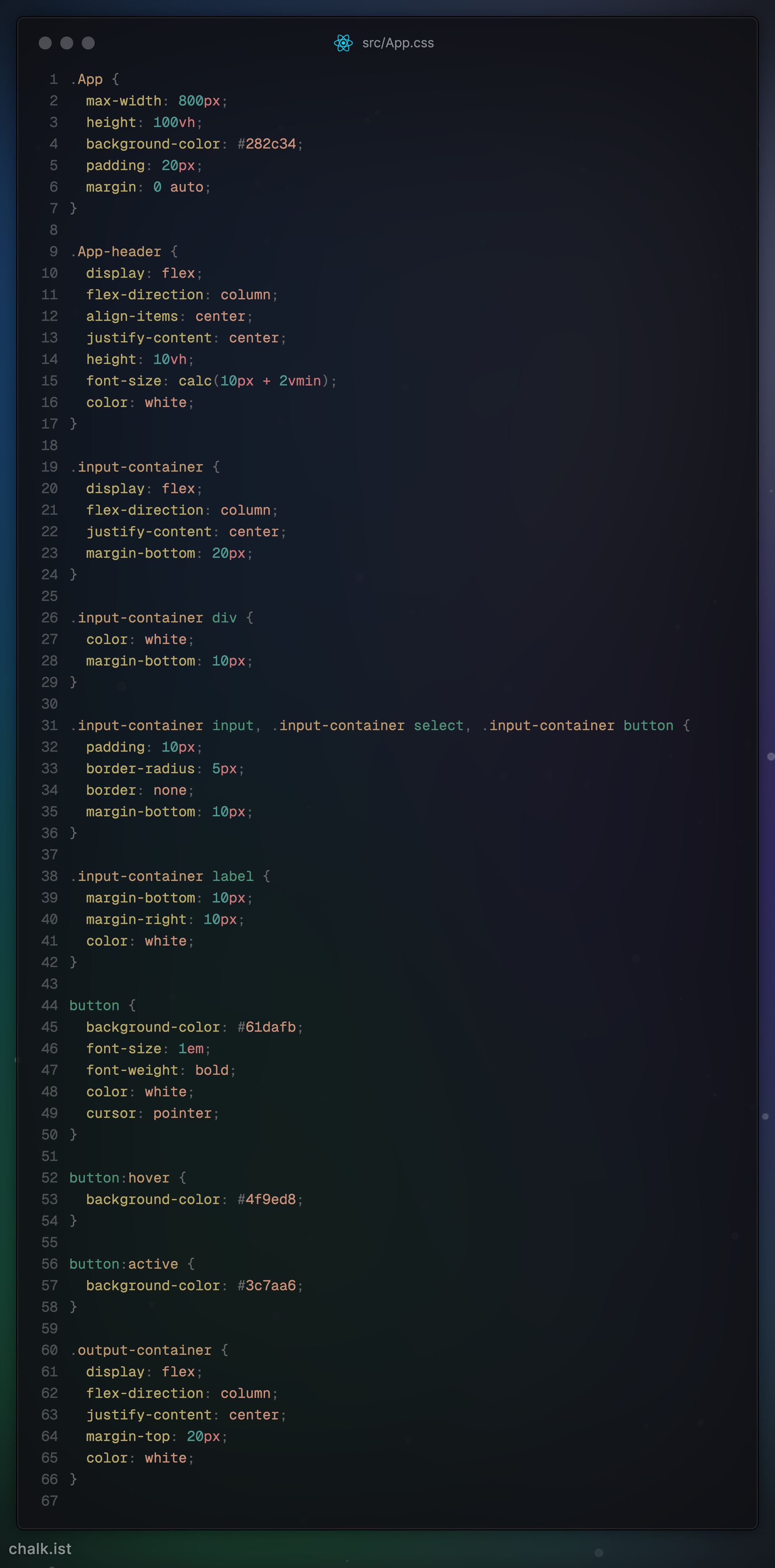
Which when we look at the app again in the browser, gives us a much better result.
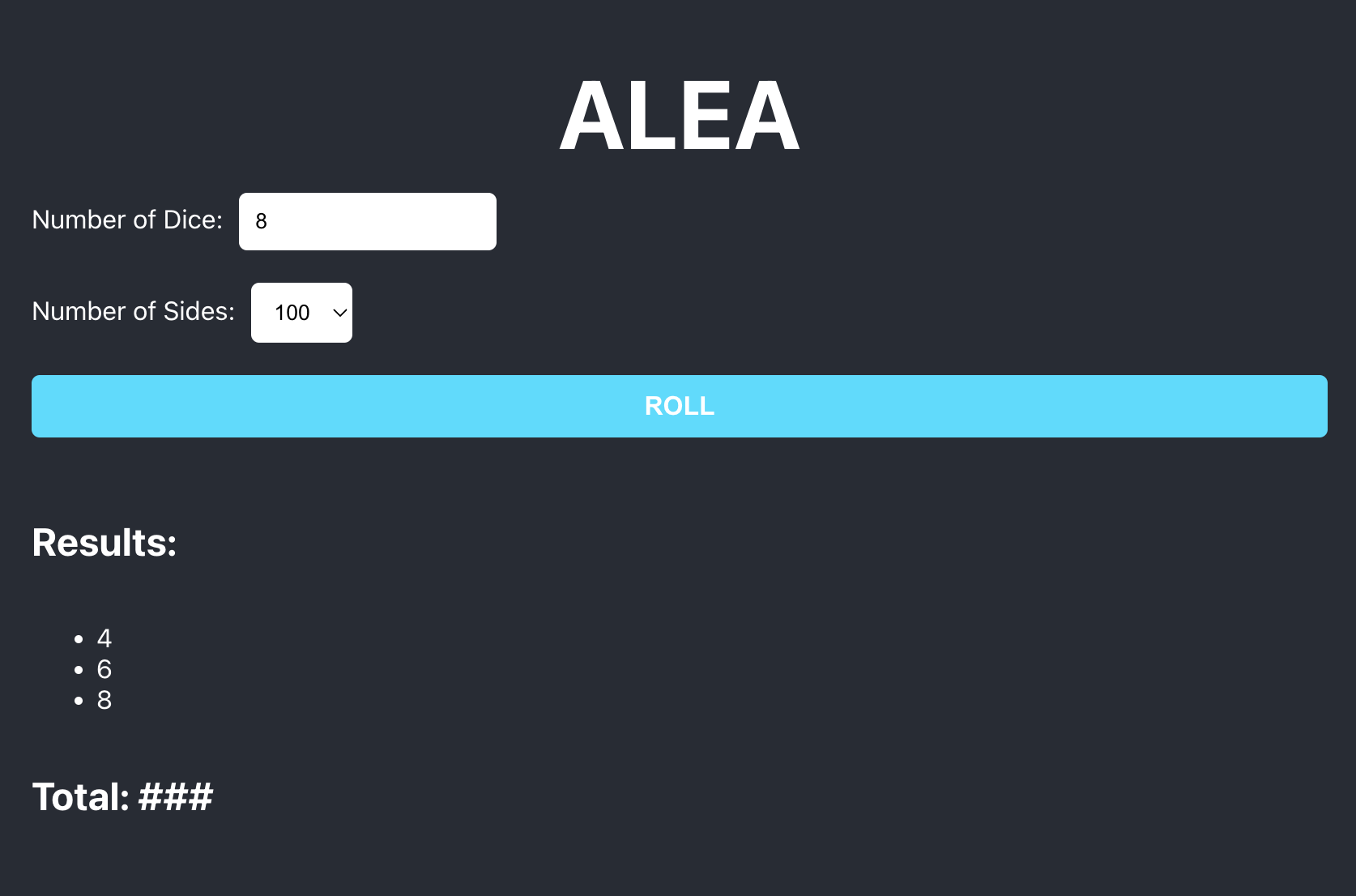
You should see the app in the middle of the screen, and it may have white columns on either side where the max-width
ends, but your screen continues. Feel free to play around with any of the styles in this file. This isn't a styling tutorial, which is worthy of a whole tutorial in and of itself. Feel free to experiment and take everything in a completely different direction as we go along - I'll be cleaning up and making things fancy between iterations. The next starting point may look different than where we finish. Just remember that functionality is essential; don't get so lost in the weeds that you can't follow along. There's always time to go wild after everything works.
Adding Reactivity
This 'works' in that you can adjust the number of dice up or down, choose different sides of the dice, and endlessly click on the button. None of it is hooked up to anything else, so let's do that. Let's go back to App.js
and add some actual React logic to the App
function before we return the JSX:
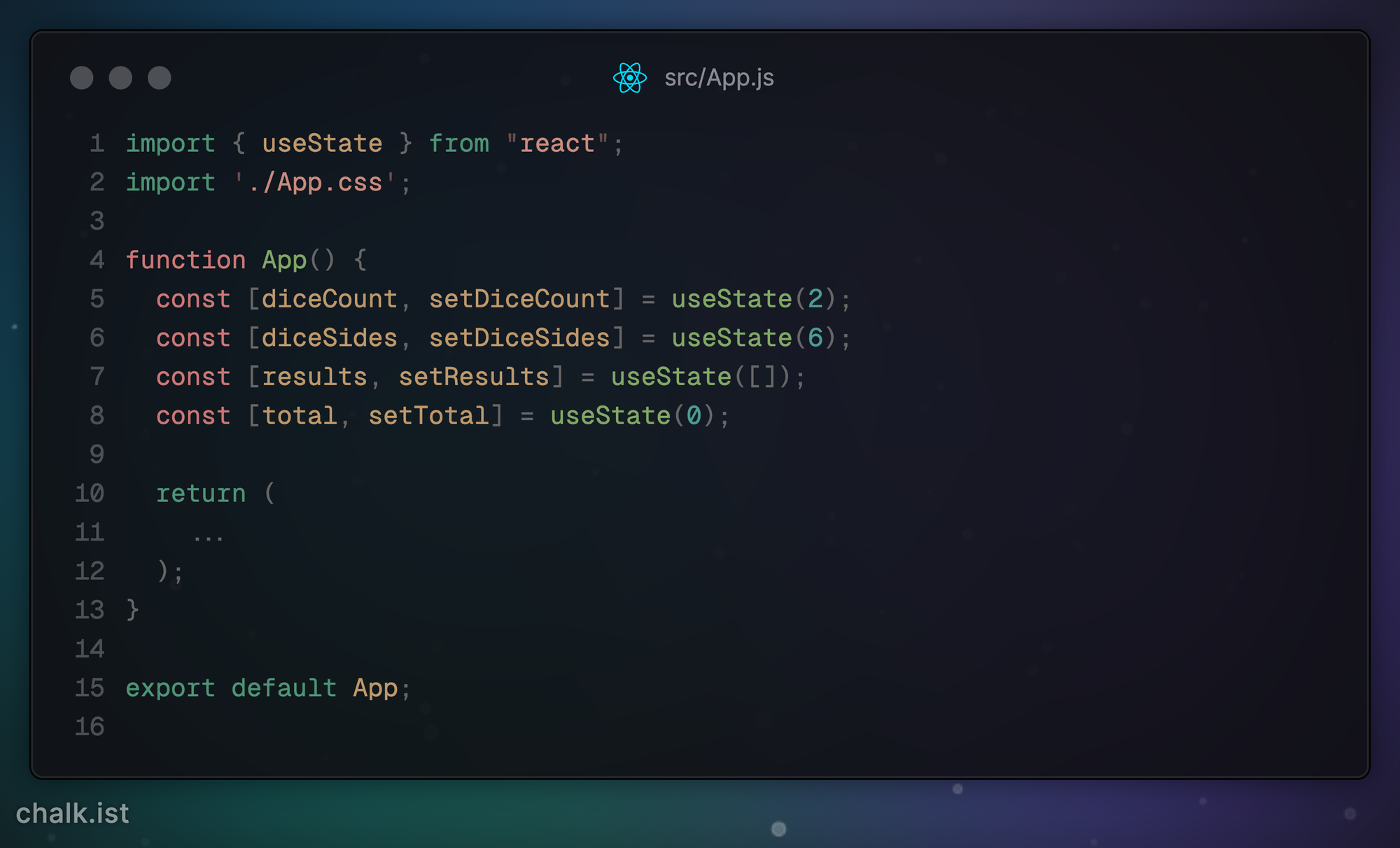
In React, you use a number of "hooks" to connect the UI (and the user) to data. useState
boils down to:
- Identifying a value we need to keep track of (the number of dice to roll)
- Saving that value somewhere we can use it and find it later for display (
diceCount
) - Exposing a function so we can change that value through our input (
setDiceCount
)
Which is what Line 5 boils down to. We then repeat that for the other values we care about in our app:
- The number of dice we roll.
- The sides (maximum value) of each of those dice.
- The set of results we get from rolling them.
- The total of our set of results.
So, we set up all of those variables and their associated functions, then apply them where needed in the UI. For example, the number of dice input needs to display the number of dice and the function to change it get applied to the number of dice input and the number of sides input:
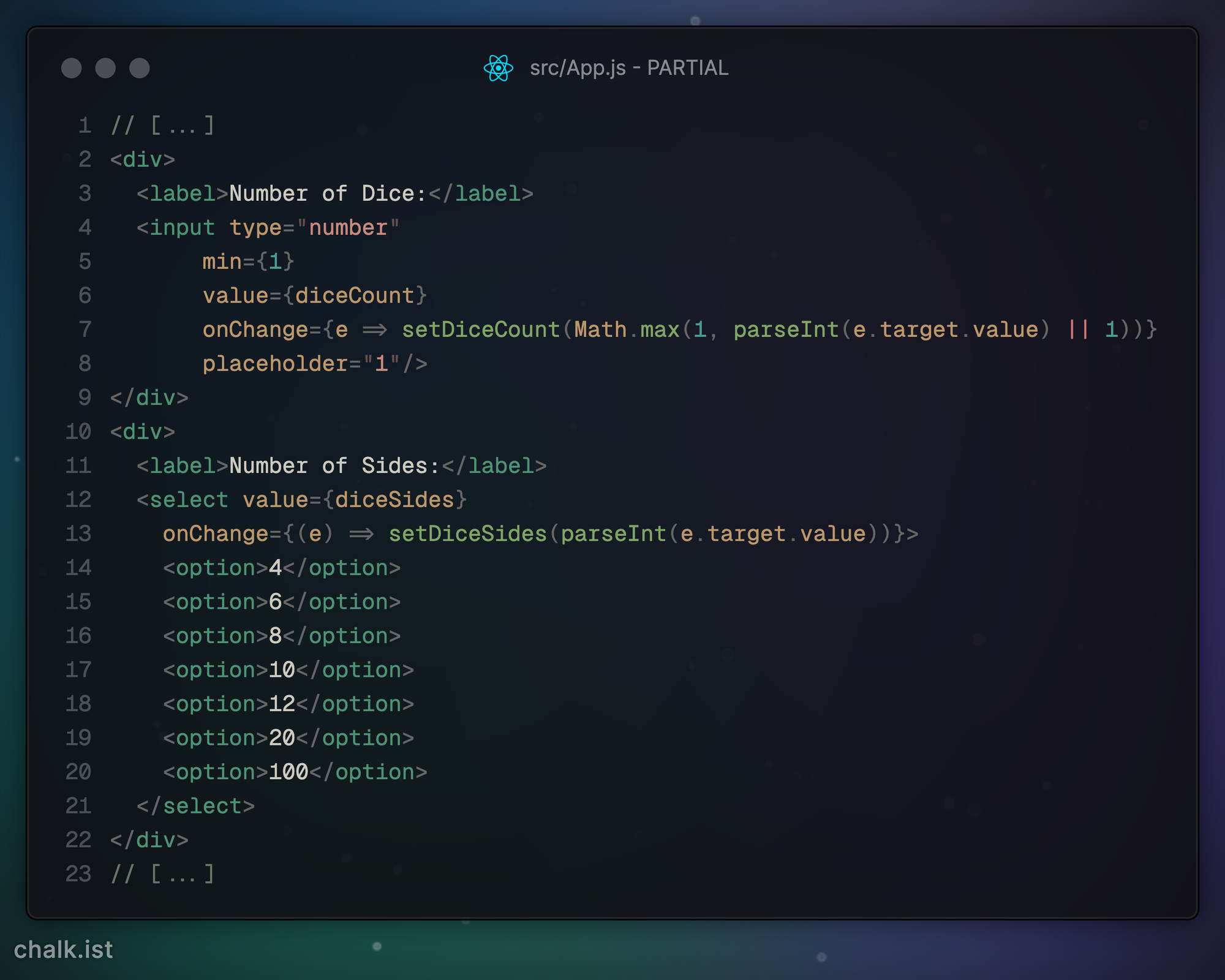
In the input section, we're assigning the number of dice input to the diceCount
variable. You can prove this works by changing the number of dice to roll in the code. On Line 5 of App.js
, set useState(2)
to useState(10)
or any other number, and check the UI again. You should see whatever value you've put in there. The same applies on Line 6 of App.js
with the number of sides.
Changing those values through the UI requires that we listen for when the input's value changes, then pass the new value to our diceCount
variable. To do that we use the onChange
hook. When that hook is called, it doesn't just get the new value – it gets the entire event associated with the user making a change. We take that event, pick out the part we need (e.target.value
) and pass that into the setDiceCount( )
function. Then we do the same thing with the diceSides
selector.
e
carries an object called target
that represents the input element the event came from. Inside the object target
is both the old value of the input before the change (previousValue
), and the value after the change, value
. That's the only part of the event we care about, so we pluck it out, and use the Math
function to make sure we get a whole number no matter what.You can read about input events here if you feel up to it.
Next, we'll hook up the ROLL button to take our input and generate the results. First, we need to make the function that does the work, putting it under the section where we declare all our variables. Hang onto your boots:
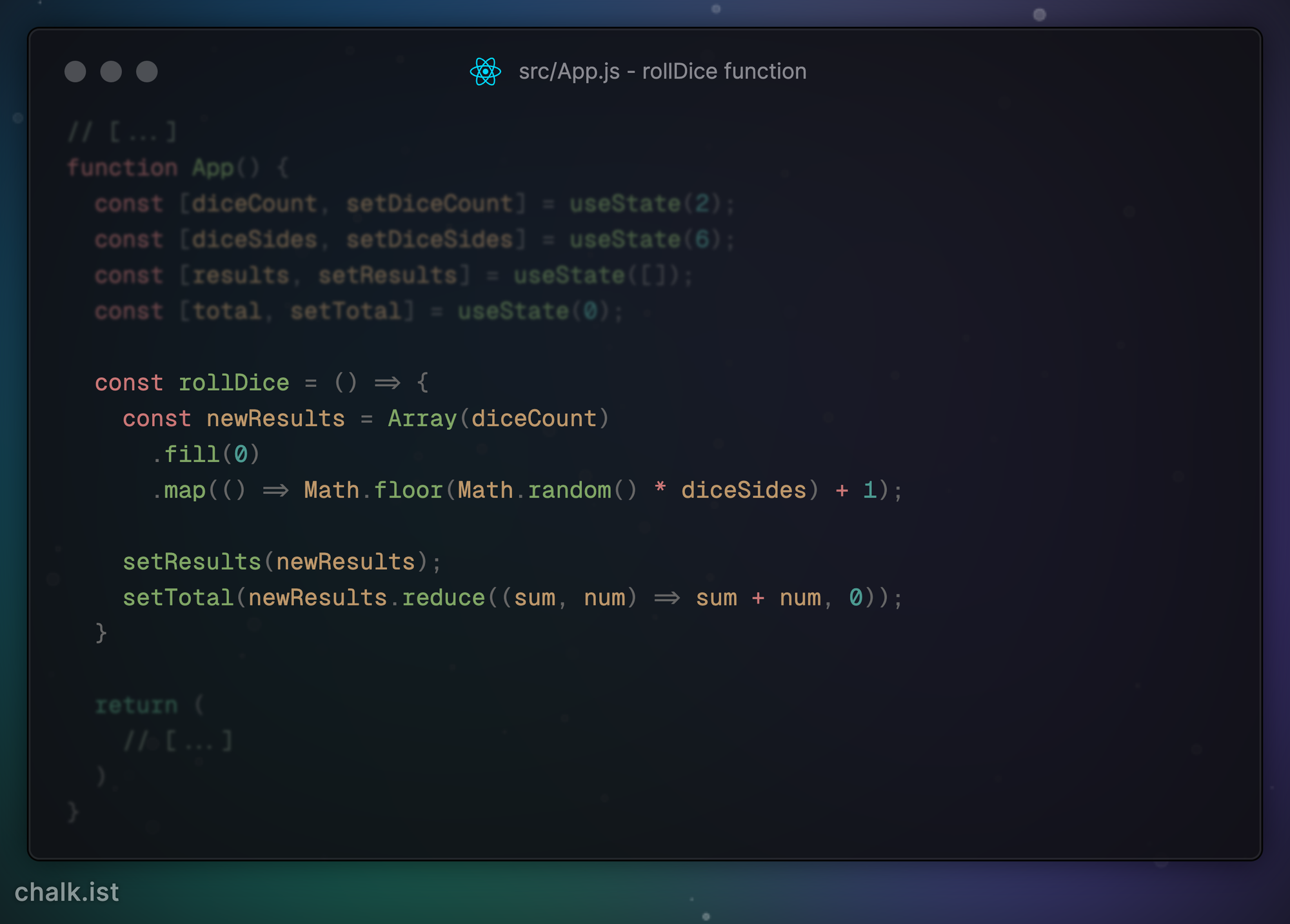
Detailed Explanation of the rollDice Function
Once we have the function written, we simply attach it to the button using the hook for the event we want to associate with it: onClick
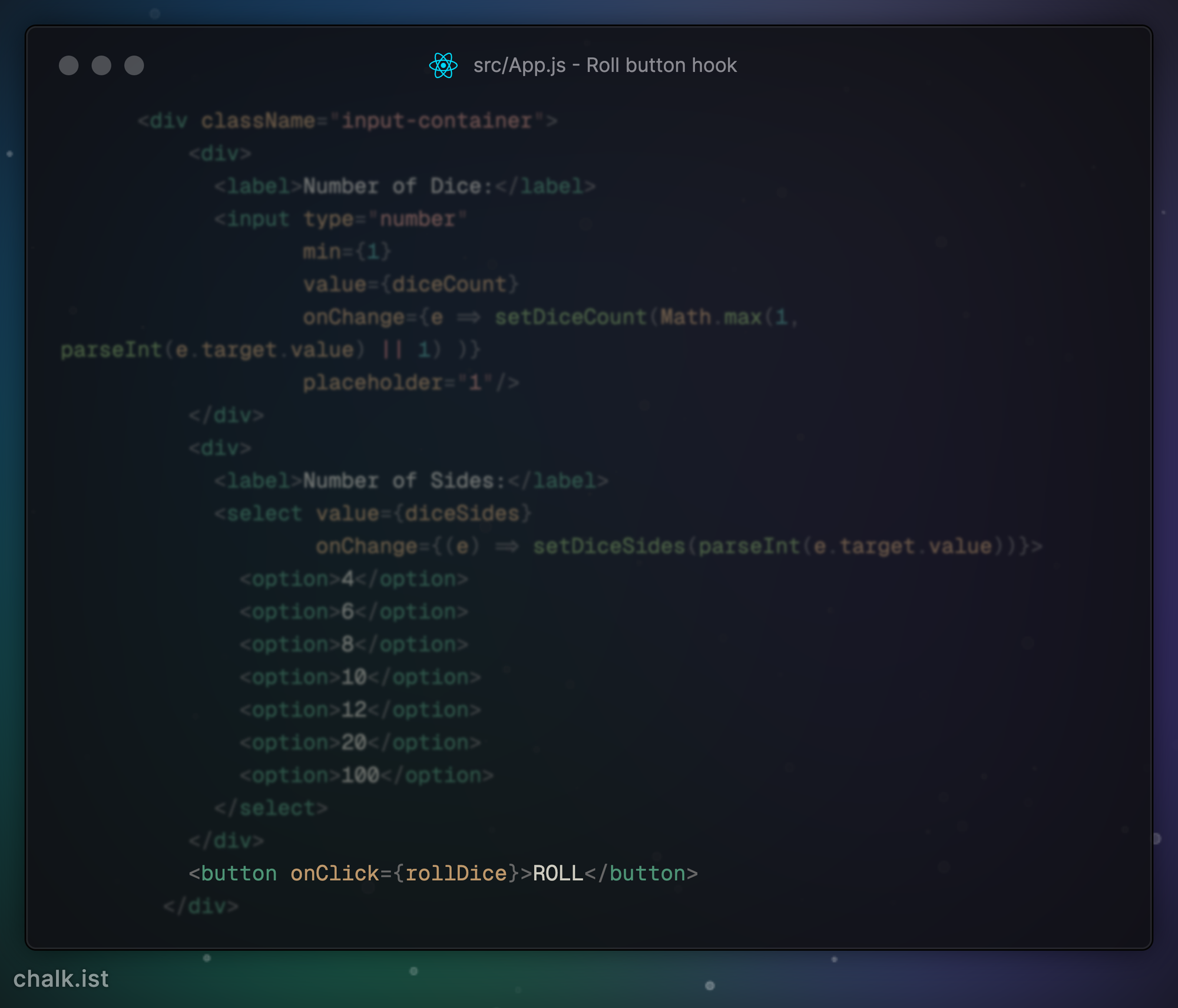
Finally, we add our output to the output section. The total is self-explanatory. For the results, remember that we have an Array of values. Arrays have a built-in function, map
, that goes through the array item by item, performing the same function for each slot. In our case, we want to take the result in that slot and make a new list-item in our list for it:
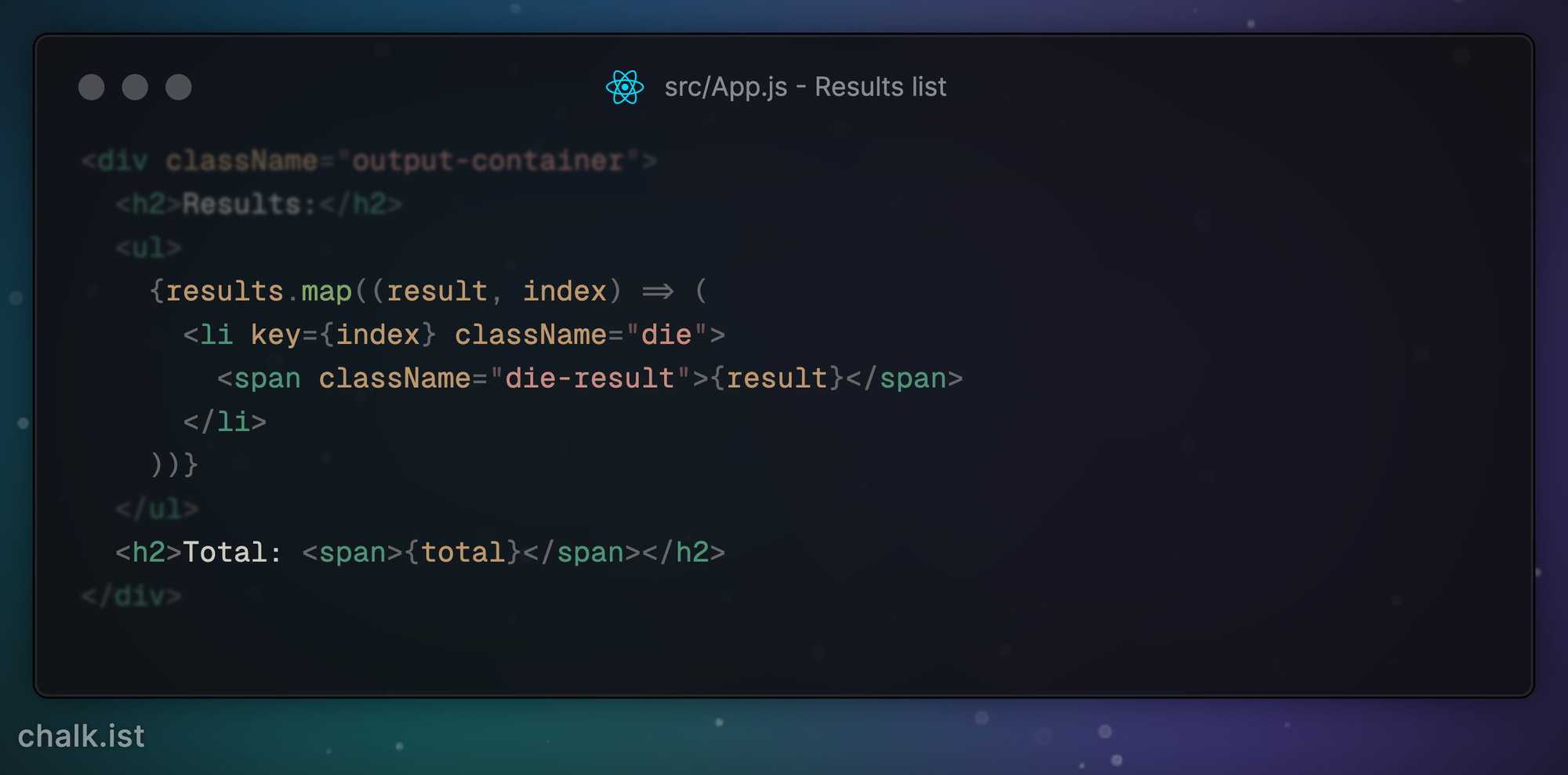
We also put the total where it belongs in the template as well.
After we're done, we check the console for any issues, fixing anything that it complains about, or giving ourselves a quick high-five if the console is clear.
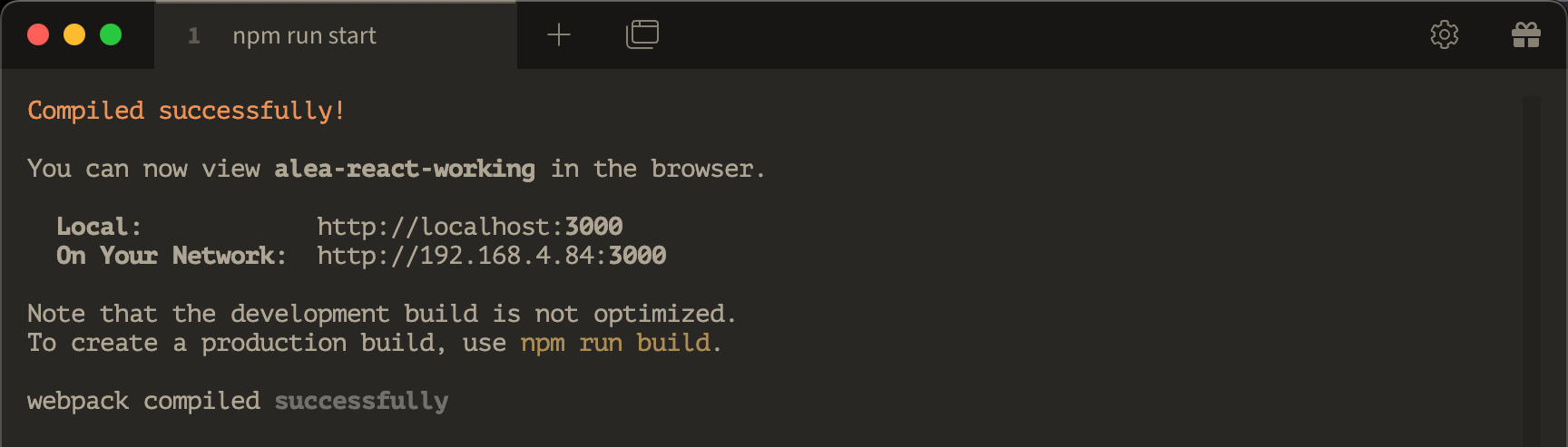
And so we can test it out in the browser:
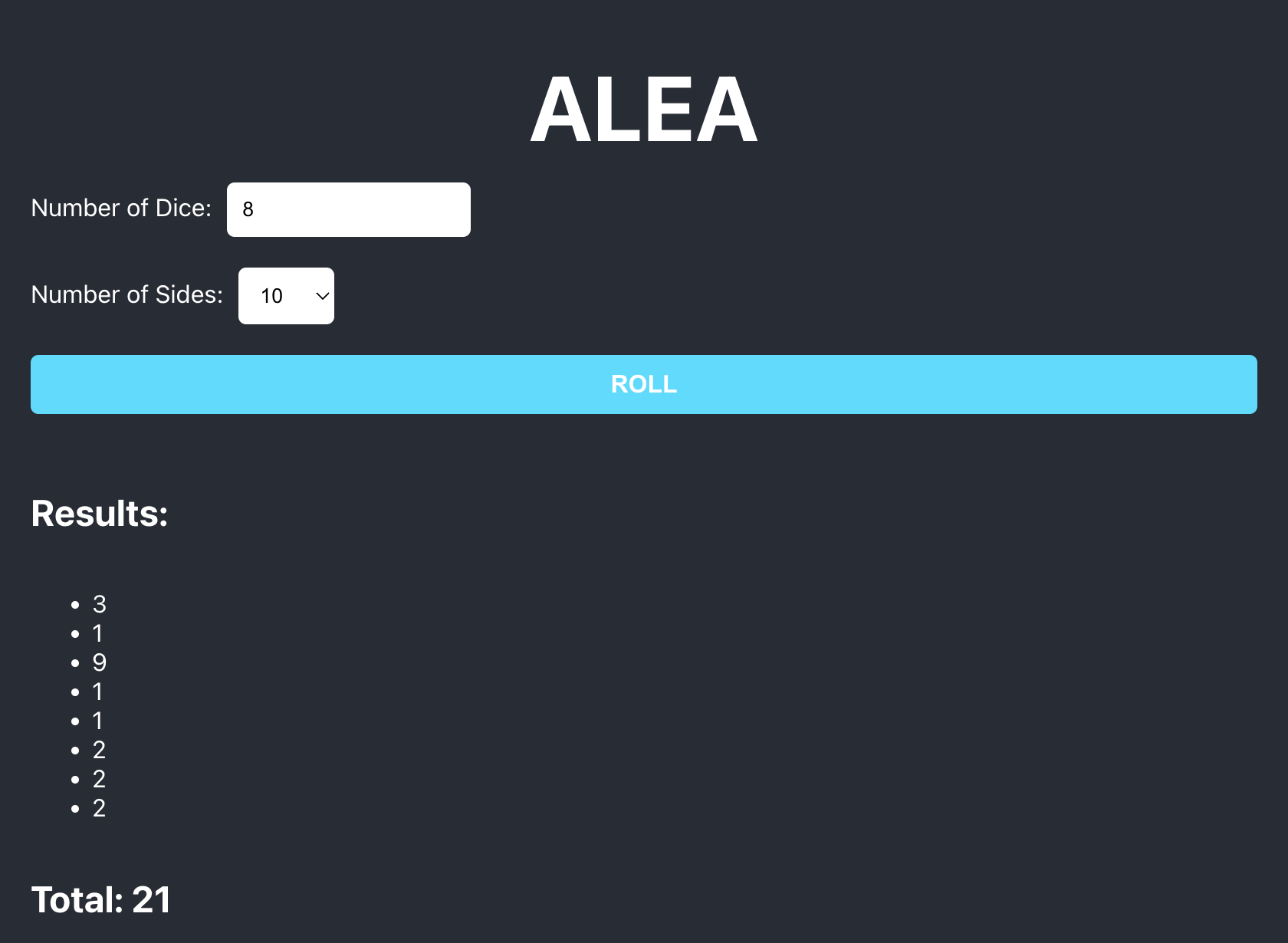
Wrapping Up
So now we've:
- Started our project in React.
- Made the basic input and output functionality.
- Styled our app so that it is not a complete eyesore.
- We tested our work to make sure it worked as expected.
Next, we will do it all over again ... in Vue!